PHP Tutorial 3 – Variables And Comments
Let’s see if we can squeeze in another tutorial before a massive asteroid smashes into the Earth. In our last mind-boggling PHP tutorial, we made our first web page using PHP. This time around, we are going to cover variables and comments.
Variables
If you’ve worked with other programming languages, or have taken a basic Algebra class, then you probably already know what a variable is. In programming, a variable is a name that can represent an assigned value. For example, we could have a variable called “X”. It doesn’t represent a value on its own, so we’ll need to assign it a value. Let’s assign the value “1” to X. Now, we have a variable “X” with an assigned value of “1”. Variables in programming aren’t limited to numbers; We could assign the phrase “Row Row Row Your Boat” to X.
In PHP, we declare a variable by simply giving a name for the variable, and setting it equal to a value. A dollar sign is placed immediately before the name of the variable as to tell the computer that the name is in fact a name for a variable, and not a function or other statement.
<html> <head> <title>My Cool PHP Page</title> </head> <body> <?php $number = 5; $words = 'Byte Revel Rocks'; $boolean = true; ?> </body> </html> |
In the code above, we declared three variables and set them equal to three different kinds of values. The first is an integer, the second is a string of text, and the third is boolean. If you are coming from another programming language, you are probably both shocked and relieved that you don’t have to declare a data type.
If you didn’t quite understand what I was saying earlier about declaring variables, you can probably follow the above variable declarations. They’re as easy as a dollar sign followed by the name of your variable, and an equal sign followed by the value you want to assign the variable. Think “$variablename = value;”.
Variables can be named with any combination of alphanumeric characters, with one exception. You can’t have a variable name that is just a number.
Depending on what kind of value you want to assign a variable, you may or may not use quotation marks around your value. Both numbers and boolean values will not have quotes around them, while strings (words) will.
What’s that? You’re not familiar with the boolean data type? It’s quite simple really. It can either be “true” or “false”.
Now that we’ve created some variables and assigned them some values, let’s go over outputting the value of a variable to the screen. You may recall that we used a function called “echo” in our last tutorial. Well, we are going to use that again to display the values of our variables.
<html> <head> <title>My Cool PHP Page</title> </head> <body> <?php $number = 5; $words = 'Byte Revel Rocks'; $boolean = false; echo $number;
echo $words;
?> </body> </html> |
The above code will output “5Byte Revel Rocks”. Similarly to how we outputted text in our last tutorial using the statement ” echo “Hello World”; “, we use the echo function followed by the name of the variable to output the value of the variable.
Take note that I didn’t echo the boolean variable. This is because the computer reads boolean values as “1” if it is true, and “0” if it is false. Using the echo function, boolean variables either result in an outputted “1” or nothing. Echo’ing boolean variables isn’t very useful.
You’re probably thinking that “5Byte Revel Rocks” is pretty ugly. Let’s stick the variables inside of a string of text to pretty them up (and make them more useful).
<html>
<head>
<title>My Cool PHP Page</title>
</head>
<body>
<?php
$number = 5;
$words = 'Byte Revel Rocks';
$boolean = false;
echo "My favorite number is $number, and $words";
?>
</body>
</html>
|
The above code will output “My favorite number is 5, and Byte Revel Rocks”. Pretty cool, eh? Including variables in strings of text is as easy as inserting the name of the variable into the string of text.
Alternatively, you can include the values of variables inside echo statements through an operation called concatenation. To do this, simply put a “.” between each string of text and and each variable. Make sure that all strings of text are enclosed by quotation marks. Like so:
<html> <head> <title>My Cool PHP Page</title> </head> <body> <?php $number = 5; $words = 'Byte Revel Rocks'; $boolean = false; echo "My favorite number is ".$number.", and ".$words;
?> </body> </html> |
Comments
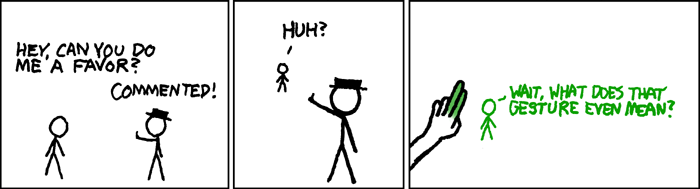
"Commented" by XKCD
In programming, comments are sections of text inside the program that aren’t run. They are marked with certain characters so that the computer knows to skip over them. They are most commonly used to add annotations, or notes, to the code. They can also be used to temporarily “mute” parts of a program.
The syntax for comments in PHP is the same as C, C++, and Perl (or rather, it allows you to use the syntax from any of those languages).
<html> <head> <title>My Cool PHP Page</title> </head> <body> <?php // this is a single-line comment # this is a single-line comment /* this is a multi-line comment */ ?> </body> </html> |
The above code will output absolutely nothing, and I believe it is pretty self explanatory.
Leave a Comment